Understanding Cardinality: Exploring Its Significance and Implementation in Python Programming
In the world of mathematics and data analysis, the term “cardinality” holds a crucial position. This concept is fundamental to understanding the uniqueness and distinctiveness of elements within a set or collection. In this article, we will dive deep into the concept of cardinality, its importance in various fields, and how to implement it effectively using Python programming.
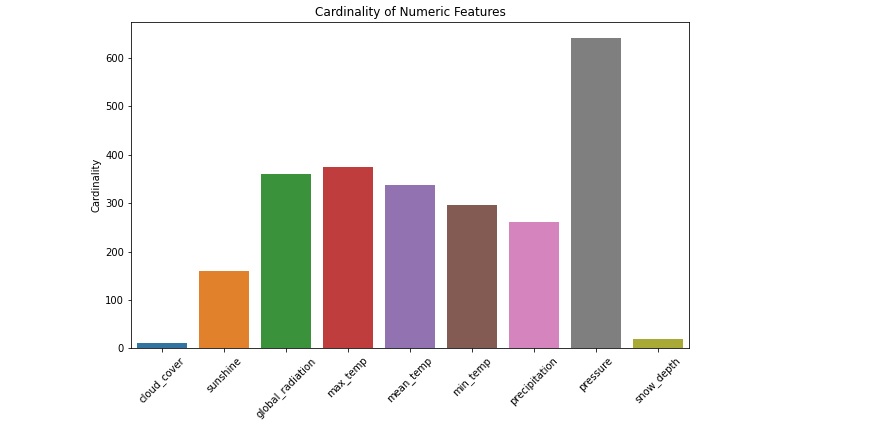
What is Cardinality?
Cardinality, at its core, refers to the count of unique and distinct elements within a set or a collection. Unlike other counting methods, it focuses solely on the presence or absence of specific items, disregarding their individual frequencies. Symbolically represented as |A|, where A is the set in question, cardinality is a foundational concept in various mathematical and analytical domains.
Importance of Cardinality
Data Analysis Insights
Cardinality plays a pivotal role in data analysis, offering insights into the uniqueness of data points. By understanding how many distinct elements exist within a dataset, analysts can identify potential outliers, anomalies, and patterns.
Database Design Considerations
In the realm of database design, cardinality defines the relationships between tables. Whether it’s a one-to-one, one-to-many, or many-to-many connection, cardinality shapes the structure of databases, influencing schema design and query optimization.
Set Theory Applications
In the broader scope of mathematics, cardinality is a cornerstone of set theory. It provides a means of comparing the sizes of different sets, including infinite sets, and aids in establishing foundational concepts in mathematics.
Calculating Cardinality in Python
Using the len()
Function
One of the simplest ways to calculate cardinality in Python is by utilizing the len()
function. By converting a collection into a set, you can obtain the count of distinct elements effortlessly.
my_list = [1, 2, 2, 3, 4, 4, 5]
cardinality = len(set(my_list))
Leveraging collections.Counter
For a more comprehensive view, the collections.Counter
class comes in handy. It not only counts the occurrences of each element but also provides the cardinality of the unique elements.
from collections import Counter
my_list = [1, 2, 2, 3, 4, 4, 5]
element_counts = Counter(my_list)
cardinality = len(element_counts)
Libraries for Efficiency
Python libraries such as NumPy and pandas offer efficient tools for calculating cardinality and handling complex data structures with ease.
import numpy as np
import pandas as pd
my_array = np.array([1, 2, 2, 3, 4, 4, 5])
unique_values = np.unique(my_array)
cardinality = len(unique_values)
my_series = pd.Series(my_array)
cardinality = my_series.nunique()
Unveiling the Significance of Cardinality
Data Profiling and Quality Assessment
Cardinality aids in data profiling, enabling analysts to assess the quality of datasets. Unusual or unexpected cardinality values can signal data issues.
Relationship Establishment in Databases
In databases, cardinality defines the connections between tables, guiding how data is linked and retrieved. It shapes the efficiency of database operations.
Foundation of Set Theory
Cardinality underpins set theory, facilitating discussions about the sizes of sets and their properties. It serves as a cornerstone of mathematical exploration.
Implementing Cardinality in Python
Step-by-Step Guide
To calculate cardinality, follow these steps:
- Convert the collection into a set to obtain unique elements.
- Use the
len()
function to count the distinct elements in the set.
Real-World Examples
Consider a dataset of customer transactions. By calculating the cardinality of the “product” column, you can determine the number of unique products sold.
Best Practices
- Be mindful of the data type and structure before calculating cardinality.
- Consider the context and purpose of the cardinality calculation.
- Utilize appropriate libraries for efficiency and accuracy.
Cardinality and Data Analysis
Detecting Duplicates
Cardinality aids in identifying duplicate entries, streamlining data cleaning processes.
Identifying Unique Elements
Calculating cardinality helps pinpoint the number of unique categories within a dataset, enabling better segmentation and analysis.
Statistical Insights
Cardinality provides insights into data distribution, which can be crucial for making informed decisions in statistical analysis.
Cardinality in Database Design
One-to-One, One-to-Many, Many-to-Many
Cardinality defines the nature of relationships between database tables. It determines how records are linked and retrieved.
Normalization and Optimization
Proper cardinality management is vital for database normalization, which reduces data redundancy and optimizes storage and queries.
Exploring Set Theory through Cardinality
Finite and Infinite Sets
Cardinality enables the comparison of set sizes, whether finite or infinite. It’s a gateway to understanding the properties of various sets.
Comparing Set Sizes
Cardinality provides a quantitative measure to compare the sizes of different sets, even when dealing with infinite sets.
Python’s Tools for Cardinality Calculation
Built-in Functions
Python’s built-in len()
function combined with set operations simplifies cardinality calculations for any collection.
External Libraries
Libraries like NumPy and pandas offer advanced capabilities for managing and analyzing data, including cardinality determination.
Challenges and Considerations
Dealing with Large Datasets
For extensive datasets, memory usage and performance should be considered when calculating cardinality.
Performance Implications
In database operations, cardinality influences query performance. High cardinality may lead to complex and slower queries.
Cardinality: Beyond Numbers
Abstract Mathematical Concept
While cardinality deals with numbers, its implications transcend into various fields, impacting analysis, design, and mathematics.
Concrete Applications
From identifying anomalies in data to optimizing database performance, cardinality finds practical applications in diverse scenarios.
Conclusion
In the realm of mathematics and data analysis, the concept of cardinality stands tall as a crucial tool. Its ability to quantify uniqueness and distinctiveness fuels insights, shapes database designs, and contributes to the foundation of set theory. By harnessing Python’s capabilities, we can seamlessly calculate cardinality and unlock its potential in various contexts.
FAQs (Frequently Asked Questions)
- Why is cardinality important in data analysis?
Cardinality helps identify unique elements in datasets, enabling outlier detection and pattern recognition. - How does cardinality influence database design?
Cardinality defines relationships between tables, affecting schema structure and query optimization. - Can cardinality be applied to infinite sets?
Yes, cardinality provides a means to compare the sizes of infinite sets, contributing to set theory. - Which Python library is useful for cardinality calculation?
Libraries like NumPy and pandas offer efficient tools for managing and analyzing data, including calculating cardinality. - What are the challenges of dealing with high cardinality?
High cardinality can lead to memory and performance issues, particularly in large datasets.